Mapping Types
Last Updated on 12. June 2023 by Mario Oettler
Mapping types assign a value to a key. The key can be any Solidity built-in value type. The value can be any type.
The syntax of a mapping declaration looks like that:
mapping(key_type => value_type) mapping_name;
assigning a value to a key is done like that:
mapping_name[key] = value;
Retrieving a value from a mapping is done like that:
retrieved_value = mapping_name[key];
Mappings always have the data location storage. They cannot be used as function parameters or return parameters for functions that are publicly visible.
If you want to retrieve a value from a mapping, you have to provide the key.
A simple representation of a mapping would be like a table, where each row has a unique key column and a value column.
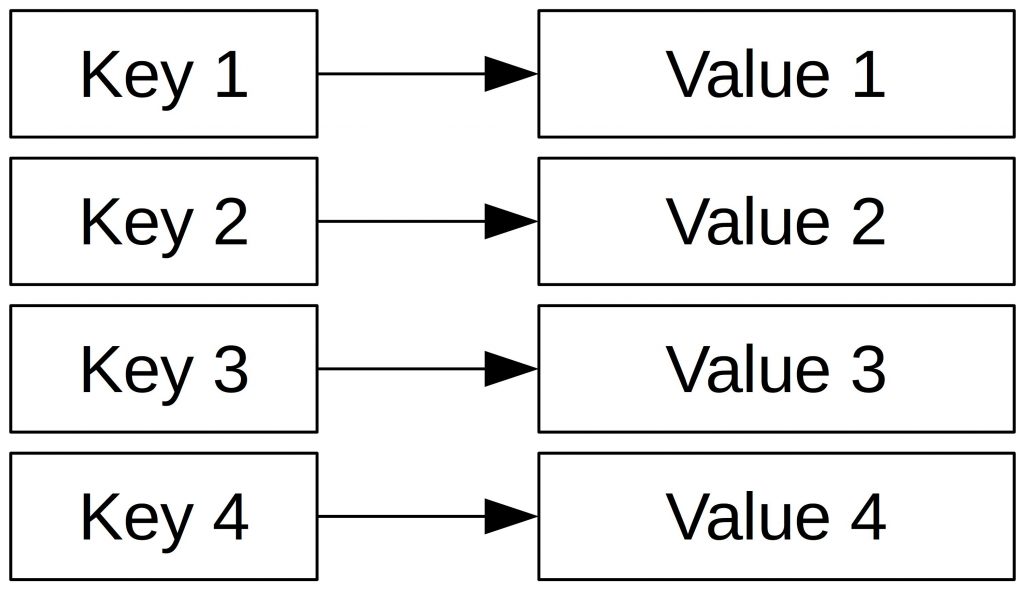
In reality, this is a bit more complicated.
Limitations of Mappings
In a mapping, the key is represented as the hash of the key. If a mapping is initialized, all possible keys exist, but their associated values are zero. The consequence is that mappings do not have a length, and it is impossible to iterate through them. It is also not possible to retrieve the keys since every key already exists.
If you want to iterate over a mapping (more precisely, values that are non-zero), you need to implement a second data structure on top of it that stores the keys. An array can serve this purpose.
You can find an example here: https://docs.soliditylang.org/en/v0.8.7/types.html#iterable-mappings
Code Example
pragma solidity 0.8.20;
contract mappingTest{
mapping(uint256 => string) public names;
function fillMapping(uint256 _key, string memory _name) public{
names[_key] = _name;
}
}
If you make a mapping public, the compiler automatically adds a getter-function. It requires the key as an input parameter. Try some “existing” keys and some non-existing keys.