Develop And Deploy A Contract
In this topic the constructor of the created contract is extended so that initially (with contract creation) tokens are minted. Then the contract is deployed in the Remix VM.
1st Step: The next step is to add function calls to the constructor. These are called once when the smart contract is created. At this point, tokens will be generated, so the mint function is called. This function was inherited via the import of the ERC1155 contract and must not be defined again in this contract (Therefore, the leading underscore). The mint function is passed four parameters:
- the address of the owner of the newly generated tokens,
- the ID of the token(s),
- the number to be minted, and
- additional data.
With the first two calls of the mint function, fungible tokens with different amounts are generated and transferred to the msg.sender, i.e., the creator of the contract. The fungible tokens have the IDs 0 and 1. In the next paragraph, three NFTs are created. Therefore exactly one token is created with each new (ascending) ID. These are also transferred to the creator of the contract. Since no data will be transferred with any mint call, the last parameter of the call can remain an empty string.
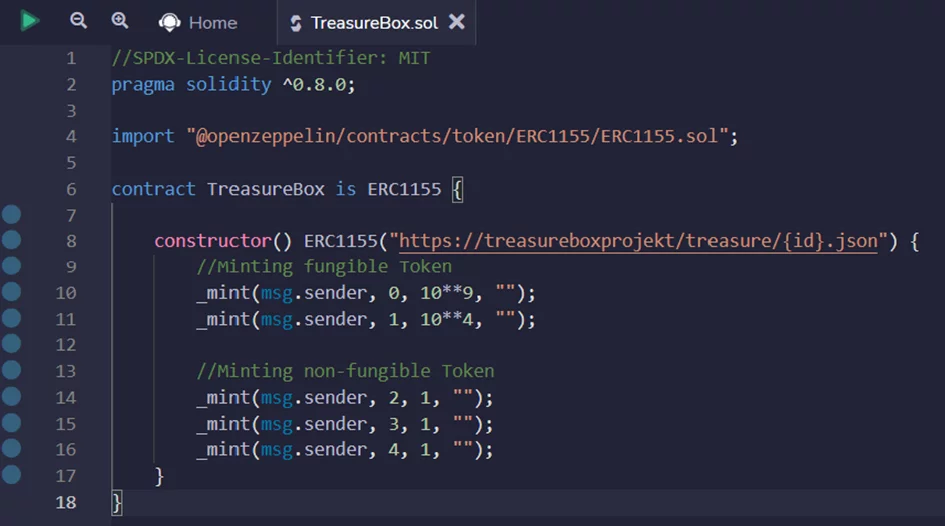
2nd Step: This should suffice as an initial basis for our contract. To evaluate the functionality, the contract must be deployed. To do this, it must first be compiled (either with shortcut CTR+S or via the Solidity Compiler tab). Remix then compiles the contract and loads the imported ERC1155 contract into the file directory. The green check mark confirms that everything could be compiled correctly. If you get an error message check your compiler version – e.g. try pragma solidity ^0.8.5 instead of ^0.8.0 or inform yourself about the newest version.
Complete Code
//SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;
import "@openzeppelin/contracts/token/ERC1155/ERC1155.sol";
contract TreasureBox is ERC1155 {
constructor() ERC1155("https://treasureboxprojekt/treasure/{id}.json") {
//Minting fungible Token
_mint(msg.sender, 0, 10**9, "");
_mint(msg.sender, 1, 10**4, "");
//Minting non-fungible Token
_mint(msg.sender, 2, 1, "");
_mint(msg.sender, 3, 1, "");
_mint(msg.sender, 4, 1, "");
}
}
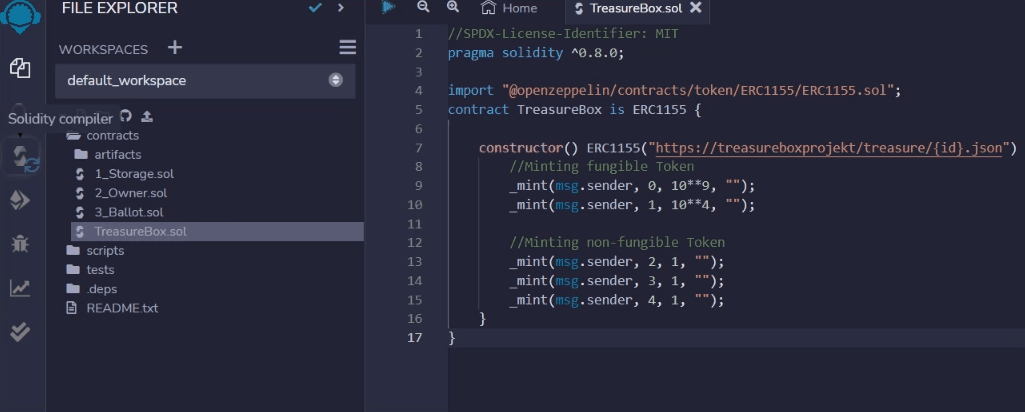
3rd Step: Initially, deploying in a virtual machine (VM) should be sufficient. In the Deploy tab of Remix, you just must select the Remix VM as the environment and the TreasureBox contract can be deployed.
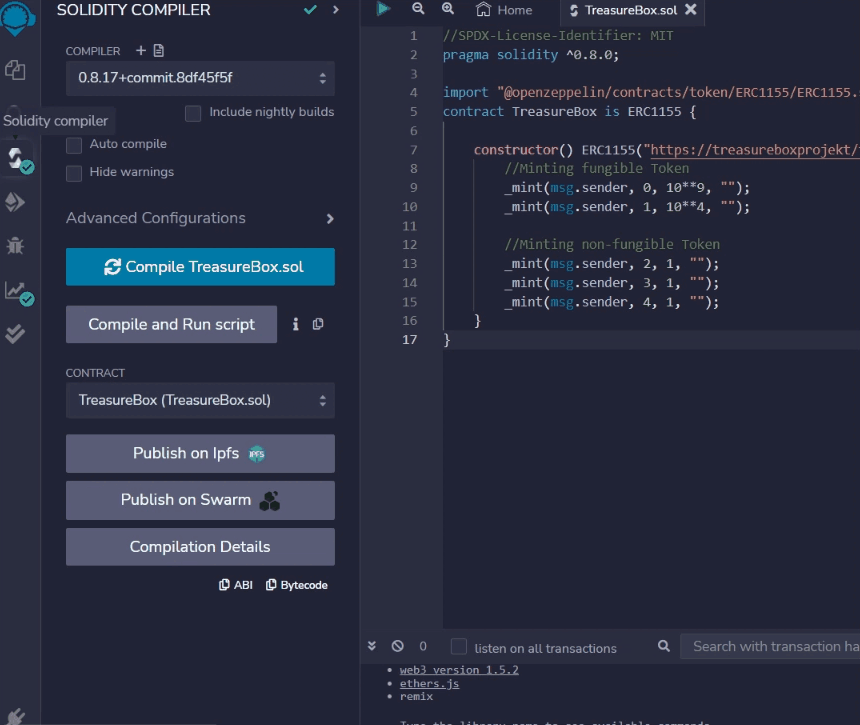
The next topic will show how to interact with the contract.