Preparation for interacting with Smart Contracts
Last Updated on 8. October 2024 by Mario Oettler
First, we set up our express server by creating a file named dapps1_server04.js. In your current folder, create another folder and add the web3.min.js.
You can use the following code.
// Here, we deliver the web3 library to the browser
// We copied the web3.min.js from the dist folder into our project.
var express = require("express");
var path = require("path");
var app = express();
app.listen(3000);
console.log("Listening to Port 3000");
app.get('/', function(req, res){
res.sendFile(path.join(__dirname, './','dapps1_browser04.html'));
app.use(express.static(path.join(__dirname, './', 'public')));
});
To interact with a smart contract, we need a smart contract. You can take this one.
pragma solidity 0.8.27;
contract DappTestContract{
struct profile{
string name;
uint256 balance;
}
mapping(address => profile) public profiles;
function payIn(string memory _name) public payable {
profiles[msg.sender].name = _name;
profiles[msg.sender].balance = profiles[msg.sender].balance+ msg.value;
}
function getMyName() public view returns(string memory){
return profiles[msg.sender].name;
}
}
Now, compile your smart contract, deploy it and copy the ABI. We will need both the address and the ABI in our JavaScript to interact with this contract. If you use the Remix IDE, you will find the ABI in the tab Solidity Compiler.
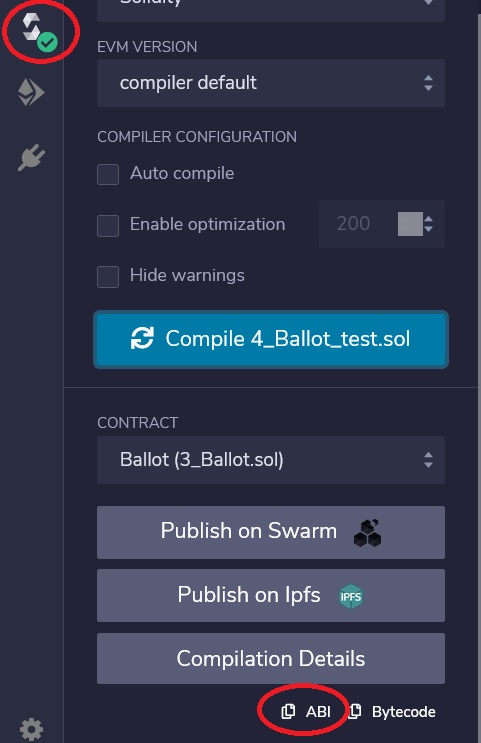